Table of Contents
- Python Strings with Examples - Studyopedia
- Python Strings with Examples - Studyopedia
- Escape Sequences in Python (with examples) - ToolsQA
- Escape Character in Python programming Language. | RebellionRider
- Escape Characters - Python: Tutorial 26 - YouTube
- #38 Python Escape Characters | Python Tutorial For Beginners - YouTube
- Escape Sequences or Characters in Python - Coding Ninjas
- Python Escape Sequences, Text, and Unicode Characters - YouTube
- PYTHON : Escape special HTML characters in Python - YouTube
- 13. Escape Characters in Python - YouTube
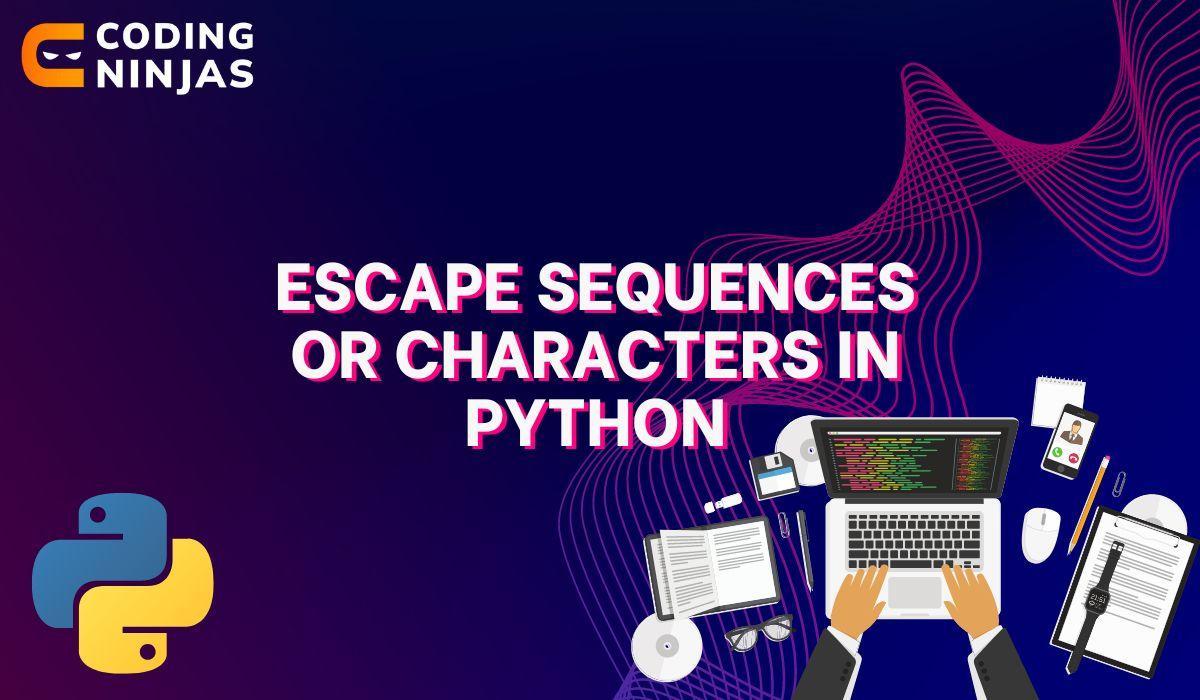

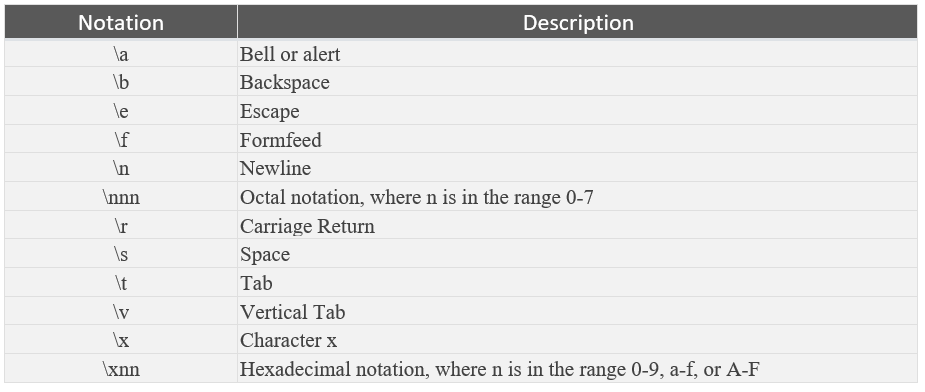
Understanding Escape Characters

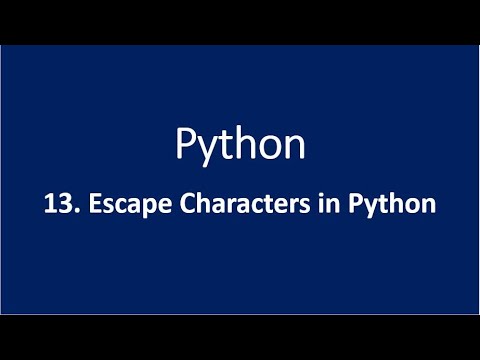
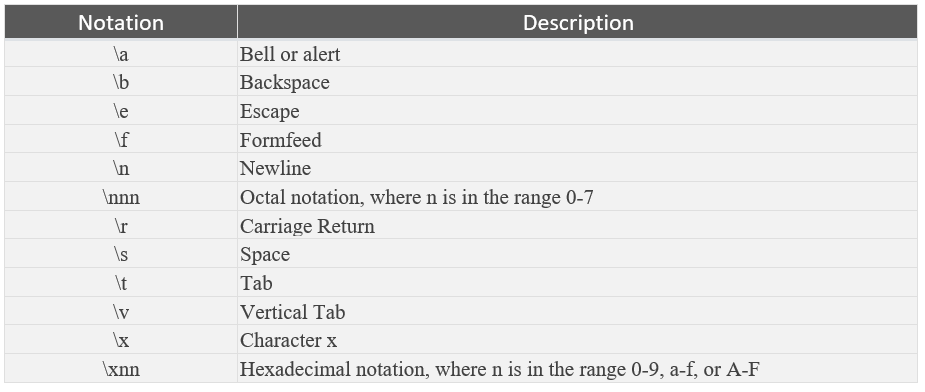
1. Using Raw Strings
One way to print escape characters is by using raw strings. Raw strings are denoted by the `r` prefix before the string. When you use raw strings, Python treats the backslash as a literal character, rather than an escape character. ```python print(r"\n") # prints \n print(r"\t") # prints \t ```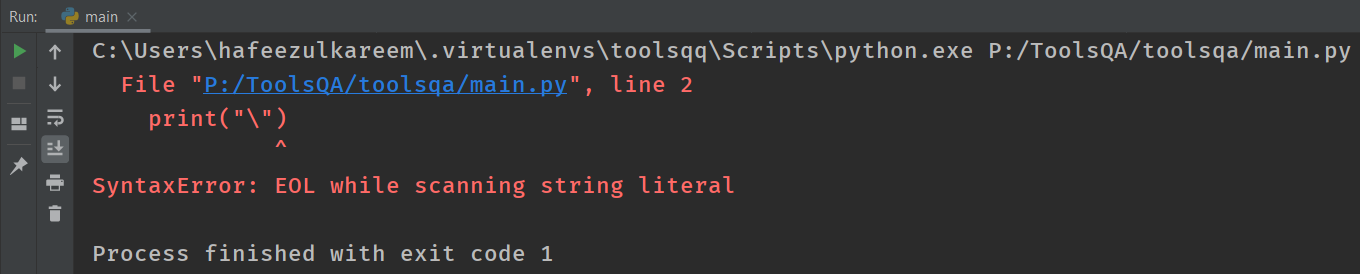
2. Using Double Backslash
Another way to print escape characters is by using double backslash (`\\`). When you use double backslash, Python treats the first backslash as an escape character, and the second backslash as a literal character. ```python print("\\n") # prints \n print("\\t") # prints \t ```
3. Using Unicode Escape Sequence
You can also use Unicode escape sequences to print escape characters. Unicode escape sequences are denoted by `\u` or `\U` followed by the Unicode code point. ```python print("\u000A") # prints newline print("\u0009") # prints tab ```
4. Using the `encode()` and `decode()` Methods
You can also use the `encode()` and `decode()` methods to print escape characters. The `encode()` method encodes the string into bytes, and the `decode()` method decodes the bytes back into a string. ```python print("\\n".encode().decode()) # prints \n print("\\t".encode().decode()) # prints \t ``` Printing escape characters in Python can be challenging, but with the right techniques, it can be made easier. In this article, we discussed four ways to print escape characters: using raw strings, double backslash, Unicode escape sequences, and the `encode()` and `decode()` methods. By mastering these techniques, developers can work with strings more efficiently and effectively.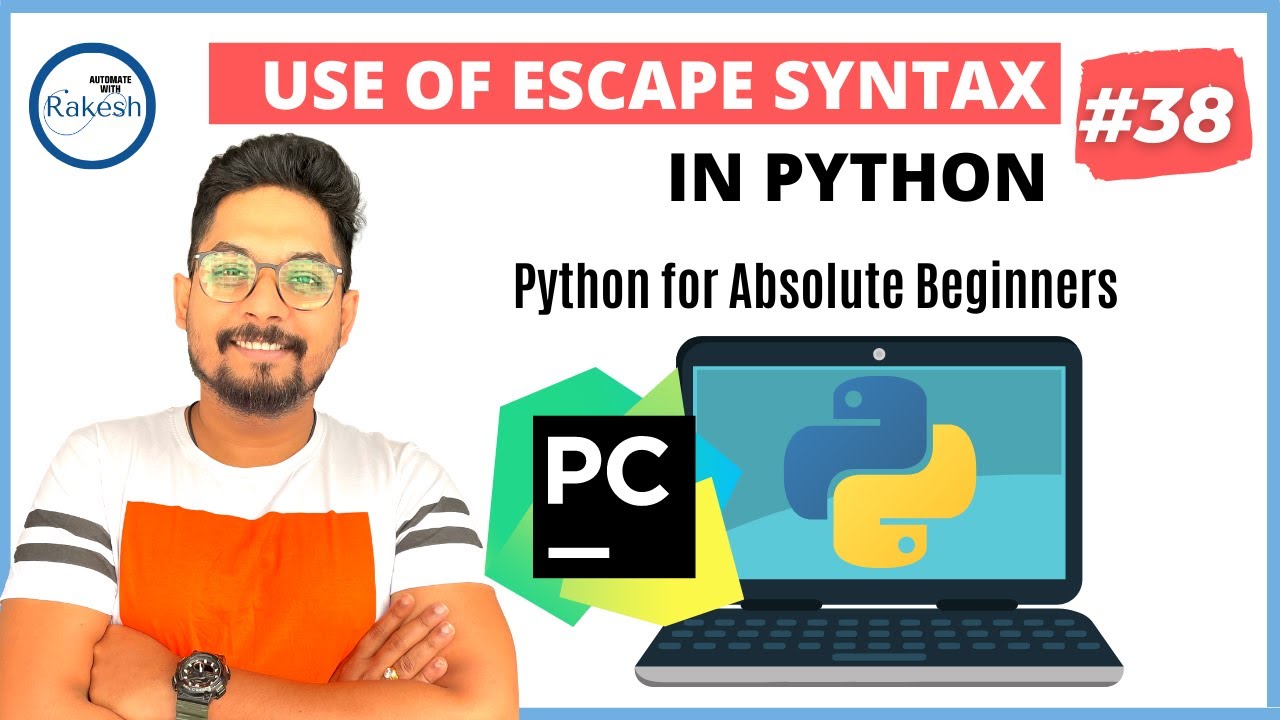